If you’re working with Python, you’ve probably come across the term pip. Pip is Python’s package manager — basically like an app store, but for Python libraries. You use it to install and manage the tools and packages your Python projects need.
Just like any other tool, pip gets updates over time. These updates can bring new features, fix bugs, or improve performance. So keeping pip up to date is actually pretty important if you want to avoid weird errors or compatibility issues.
In this post, I’ll walk you through how to check your pip version and how to update it properly.
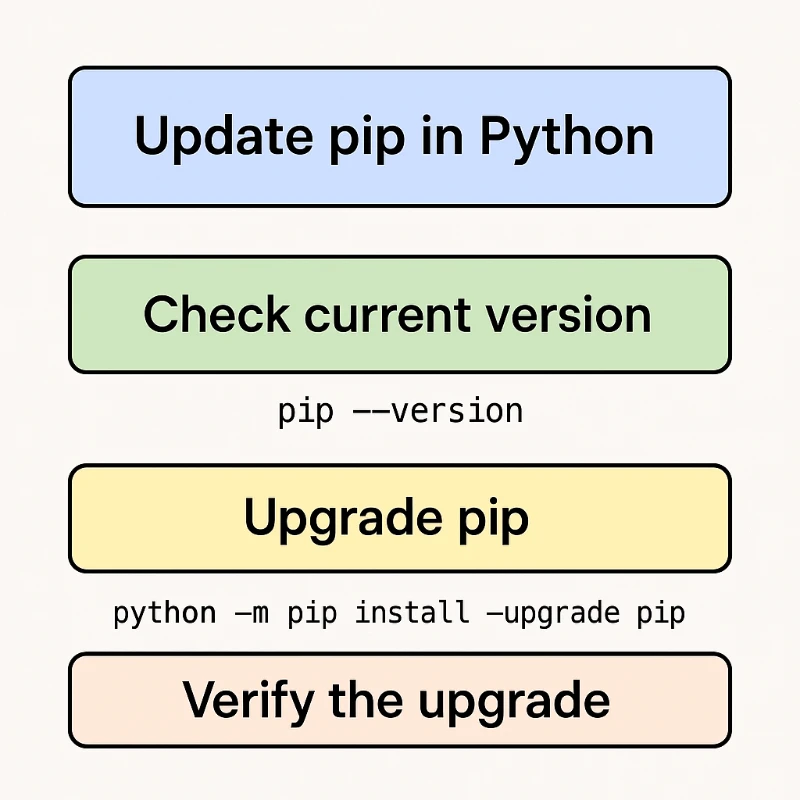
What Is Pip?
Pip stands for “Python Installer Package.” It’s the command-line tool that helps you install external Python packages.
Let’s say you want to use the requests
library in your project. All you have to do is run this in your terminal:
pip install requests
And pip downloads and installs it for you. Easy. But if pip itself is outdated, you might start seeing errors when trying to install or use certain packages.
How to Check Your Pip Version
First, you might want to see which version of pip you’re using. Just run this in your terminal:
pip --version
You’ll see something like this:
pip 23.0.1 from /usr/lib/python3.10/site-packages/pip (python 3.10)
This tells you both your pip version and which Python version it’s tied to. If the version looks old, time for an update.
How to Update Pip
This is the go-to command to upgrade pip:
python -m pip install --upgrade pip
Here’s what it does:
- Runs Python (
python -m
) - Calls the pip module
- Tells it to install a newer version of pip
After it runs, pip should be updated to the latest version available. You can check again with pip --version
.
If You're Using Python 3
Depending on your system, you might have both Python 2 and Python 3 installed. In that case, use this instead:
python3 -m pip install --upgrade pip
That way, you’re making sure pip tied to Python 3 is the one being updated.
Updating Pip Inside Virtual Environments
If you’re using virtual environments (which you totally should for Python projects), keep in mind that each environment has its own pip.
So even if you updated pip globally, your virtual environment might still be using an older version. To fix that, activate the virtual environment first, and then run the same upgrade command:
python -m pip install --upgrade pip
Now your virtual environment is up to date too.
What Happens If You Don't Update Pip?
Old versions of pip might not work well with newer Python libraries. For example, you might see this kind of error when trying to install something:
ERROR: Could not find a version that satisfies the requirement ...
In many cases, the fix is as simple as updating pip. So rather than spending hours Googling error messages, updating pip could save you a lot of time.
Conclusion
Keeping pip updated is one of those little things that can save you from big headaches. So yeah, updating pip takes like 10 seconds — but can prevent hours of frustration.
Comments (0)
Sign in to comment
Report